In this tutorial, we will learn what is ‘element not interactable exception’ and How to resolve this exception.
In Selenium, this is common to face any exception while finding the web element. In all of that exception most common exception is element not interactable exception which we are going to discuss in this tutorial.
Table of Contents
What is ‘element not interactable’ exception in selenium? OR
[exception in thread “main” org.openqa.selenium.elementnotinteractableexception: element not interactable]When a web element is present in the HTML DOM but it is not in the state that can be interacted. In other words when element is found but we can’t interact with it, then it throws ElementNotInteractableException.
Reason behind this exception: –
“element not interactable” exception may occur due to various reason.
- Element is not visible
- Element is present in off screen (After scroll down it will display)
- Element is present behind any other element
- Element is disable
Let’s replicate the same scenario and then will solve it.
Scenario:
Open google.com
Type “Facebook” in google search box
Click on first auto suggestion option
Verify title of the page
Selenium Script:
package datapkg;
import java.util.concurrent.TimeUnit;
import org.junit.Assert;
import org.junit.Test;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class ElementNotInteractable {
static WebDriver driver = null;
public static void main(String[] args) throws InterruptedException {
System.setProperty("webdriver.chrome.driver", "C:\\Users\\AJEET\\Downloads\\chromedriver_win32\\chromedriver.exe");
driver = new ChromeDriver();
driver.manage().window().maximize();
driver.manage().timeouts().implicitlyWait(30, TimeUnit.SECONDS);
driver.get("https://www.google.com/");
driver.findElement(By.name("q")).sendKeys("Facebook");
Thread.sleep(2000);
driver.findElement(By.xpath("//div[@class='UUbT9']/div[2]/ul[1]//following::span[1]")).click();
Assert.assertTrue("facebook - Google Search", true);
}
}
Output screenshot: –
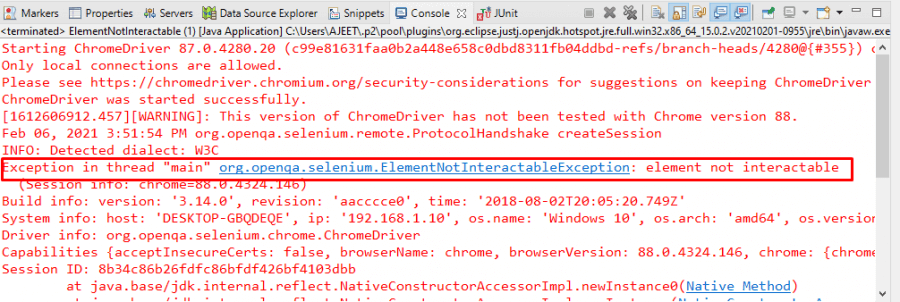
Now I will write the same script with wait command.
I will put wait command after typing “Facebook” in Google search.
package datapkg;
import java.util.concurrent.TimeUnit;
import org.junit.Assert;
import org.junit.Test;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class ElementNotInteractable {
static WebDriver driver = null;
public static void main(String[] args) throws InterruptedException {
System.setProperty("webdriver.chrome.driver", "C:\\Users\\AJEET\\Downloads\\chromedriver_win32\\chromedriver.exe");
driver = new ChromeDriver();
driver.manage().window().maximize();
driver.manage().timeouts().implicitlyWait(30, TimeUnit.SECONDS);
driver.get("https://www.google.com/");
driver.findElement(By.name("q")).sendKeys("Facebook");
Thread.sleep(2000); // Put this sleep command.
driver.findElement(By.xpath("//div[@class='UUbT9']/div[2]/ul[1]//following::span[1]")).click();
Assert.assertTrue("facebook - Google Search", true);
}
}
This test script runs successfully.
Must Read: How To Handle Tooltip in Selenium Webdriver
This exception “ElementNotInteractableException” may occur in many scenarios when an element is not visible or element is disable or element is nested. Below are the some other solution to resolve this problem.
Other Solutions: –
1. If element is not visible then wait until element is visible. For this we will use wait command in selenium
WebDriverWait t = new WebDriverWait(driver, timeout);
t.until(ExpectedConditions.visibilityOf(element));
t.until(ExpectedConditions.elementToBeClickable(element));
2. If element is in off screen then we need to scroll down the browser and interact with element.
We will use JavaScriptExecutor interface that helps to execute JavaScript method through Selenium Webdriver.
JavascriptExecutor Js1 = (JavascriptExecutor) driver;
Js1.executeScript("window.scrollBy(0,1000)"); //scroll 1000 pixel vertical
People also ask:
1. How do you check an element is not present in selenium?
Using below commands we can verify element presence on page or not.
verifyElementPresent – It will return true if element present on the page otherwise return false.
verifyElementNotPresent – It will return true if element not present on the page otherwise return false.
2. Is element visible selenium?
Using “isDisplayed” command we can verify an element is visible or not.
If element is visible on the page then it returns true, otherwise return false.
3. Can Selenium interact with hidden element?
By default selenium does not interact with hidden element and throws ElementNotVisibleException but by using javaScript executor interface we can handle hidden element.
4. How do you check a button is enabled?
Using “isEnabled()” command we can check.
Summary:
In this post, we have covered what is ‘element not interactable’ exception and ‘How to handle element not interactable’ exception in selenium.
Also added some additional questions related exception in selenium. I am sure this content added some additional value in your skills and also helpful to preparation of your interviews.
Final word, Bookmark this post “How To Resolve Element Not Interactable Exception” for future reference.
If you have other questions or feedback, the comment section is yours. Don’t forget to leave a comment below!
Must Read Below Post: –