In this post, we will learn what are the common exceptions found in Selenium and how we can handle this.
Getting an exception in automation script is very common. ‘Exception’ means abnormal conditions or events which are occur in the programming and disrupts the normal flow of program execution.
In Automation scripts, exception found due to synchronization, web-element not found, incorrect syntax, parameters etc.
Table of Contents
Common Exceptions in Selenium WebDriver:
There are different exceptions found in Selenium. Below are few Selenium exceptions faced while running a test.
ElementNotVisibleException – If element is present in the DOM but visibility is off, It means element is hidden. If Duplicate Xpath is found by selenium. It means more than one elements has same xpath. If synchronization problem(If selenium is faster than application or vice versa.) occur then this exception thrown.
NoSuchElementException – If element is not present in the DOM or we have given the wrong locator of the element or element is present in any frame and we haven’t switched that frame then selenium throw this exception.
NoSuchWindowException – If the window target to be switch does not exist.
NoSuchFrameException – If the frame target to be switched to does not exist.
NoSuchAttributeException– If the desired attribute does not exist.
NoAlertPresentException – If the target alert is unavailable.
ElementNotSelectableException – An element is disabled (can not be clicked/selected) in spite of being present in the DOM.
ElementNotInteractableException – If an element is present in the DOM but not able to intractable.
JavascriptException – This issue occurs while executing JavaScript.
StaleElementReferenceException – When Selenium trying to interact with an element at that time page got refreshed or ajax calls happened then selenium throw this exception.
(Internally when selenium interacting the element it creates a random ID by using this selenium interact with the element but if page got refreshed or ajax calls happened then ID is changed, now that ID is stale from the page, So selenium throw this exception.)
SessionNotFoundException – A new session could not be created.
TimeoutException – When a command takes longer time than given wait time to avoid the ElementNotVisibleException.
WebDriverException – When selenium trying to interact with a web element after the browser is closed.
Handling Selenium Exceptions:
There are few ways to handle the exceptions in Selenium.
Try – catch block – Using this block we can handle the exceptions. The code which can cause of exception put this into try block and we will handle the exception in catch block.
Syntax –
try { // Some code } catch(Exception e) { // Code for Handling the exception }
Multiple Catch block – We can use multiple catch block with one try block. Means we can handle multiple exceptions or every type of exceptions at the same time. There is no limitation on number of catch blocks.
Syntax –
try { //Code } catch (Exception1 e1) { //Code for Handling Exception1 } catch (Exception1 e2) { //Code for Handling Exception2 }
Throw – Throw keyword is used to throw the exception to handle it at the run time.
Syntax –
public static void Function() throws Exception { try { // write your code here. } catch (Exception e1) { // Do whatever you wish to do here // Now throw the exception back to the system throw(e1); } }
How To Handle element not found exception in Selenium WebDriver
There are different reasons for this exception like –
If element is not present in the DOM or Synchronization issue or we have given the wrong locator of the element or element is present in any frame and we haven’t switched that frame then selenium throw this exception.
Handling –
Synchronization issue – if the reason is synchronization related use explicit wait command to handle this exception.
Syntax –
WebDriverWait wait = new WebDriverWait(driver, 3); WebElement ele = wait.until(ExpectedConditions.visibilityOfelementLocated(locator));
Read Selenium basics
How To Handle stale element exception in Selenium:
Stale –>Not Fresh, Old
Reason of this exception: – If the page changes or reloads, DOM is rebuilt. Previously found elements become stale.
Handling –
- Always find the element when you need it or
- Put the statement in try-catch block which are throwing this exception.
Selenium Webdriver Exception Hierarchy: –
There are two types of exceptions.
- Checked Exceptions
- Unchecked Exceptions
Selenium exceptions come under unchecked exceptions (Runtime exceptions).
We will show here runtime exceptions hierarchy.
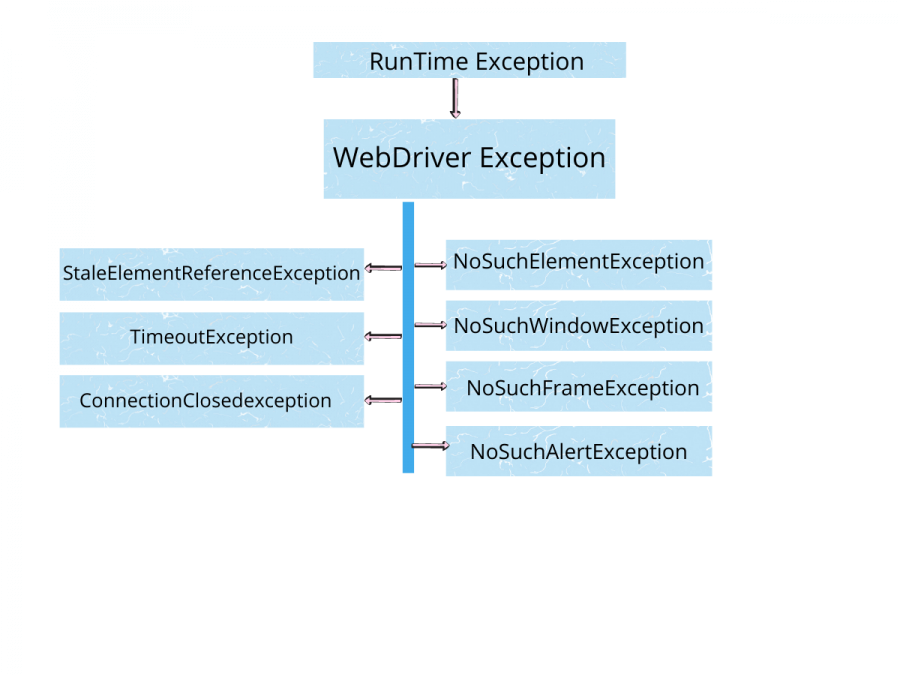
Checked Exception in Selenium:
Checked exception is also known as compile time exception that must be either caught or declared in the method.
Checked exceptions are subclasses of Exception class.
Example of checked exception: ClassNotFoundException, IOException, SQLException, FileNotFoundException.
Summary:
In this post, we have covered ‘Different Exceptions in Selenium WebDriver’.
We have also learnt ‘How to handle these exceptions in Selenium’.
I am sure this content added some additional value in your skills and also helpful to start selenium from scratch.
Final word, Bookmark this post ‘Different Exceptions in Selenium WebDriver’ for future reference.
If you have other questions or feedback, the comment section is yours. Don’t forget to leave a comment below.